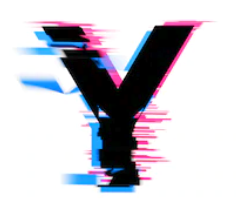
Note taken from “freeCodeCamp.org” YouTube video.
Crouse developed by Mike Dane from mikedane.com.
Basic
Data Type
- INT
- DECIMAL(a,b) a: total digits b: digits after ‘.’
- VARCHAR(N)
- BLOB
- DATE
- TIMESTAMP
Set Up Table
Image from “freeCodeCamp.org” YouTube video
Create Table
1 | CREATE TABLE student ( |
or
1 | CREATE TABLE student ( |
Print Table Data
SELECT * FROM student;
Constraints
AUTO_INCREMENT
Don’t need to specify that argument and give value, it will increase the value automatically
NOT NULL
Need to be initial
UNIQUE
For that column, no two rows have the same data
DEFAULT
1 | CREATE TABLE student ( |
Describe Table
DESCRIBE student;
Delete Table
DROP TABLE student;
Modify Table
Add column:
ALTER TABLE student ADD gpa DECIMAL;
Delete column:
ALTER TABLE student DROP COLUMN gpa;
Insert
INSERT INTO student VALUES(1, 'Jack', 'Biology');
If don’t know some arguments’ value:
INSERT INTO student(student_id, name) VALUES(1, 'Jack');
Update
1 | UPDATE student |
OR/AND keyword, Set multiple
1 | UPDATE student |
Other comparision options: =, <>, >, <, >=, <=
Update all row
1 | UPDATE student |
Delete
Delete all rows
1 | DELETE FROM stduent; |
Delete specified rows
1 | DELETE FROM stduent |
Basic Queries
SELECT
Select all
1 | SELECT * |
Select specified columns
1 | SELECT name, major |
or
1 | SELECT stduent.name, student.major |
Select by order (column)
Ascending by default (ASC)
1 | SELECT stduent.name, student.major |
Descending order (DESC):
1 | SELECT stduent.name, student.major |
More order
If name the same, further order by id
ORDER BY name, id
LIMIT
Only get two rows
1 | SELECT * |
IN
1 | SELECT * |
Advance
Image from “freeCodeCamp.org” YouTube video
Code
1 | CREATE TABLE employee ( |
Queries
Select all employees
1 | SELECT * FROM employee; |
Select all exployees ordered by salary
1 | SELECT * |
Select all exployees ordered by sex then name
1 | SELECT * |
Select first 5 exployees
1 | SELECT * |
Select first and last name of all exployees
1 | SELECT first_name, last_name |
AS keyword
Name a column differently
Select the forename and surnames names of all employees
1 | SELECT first_name AS forename, last_name AS surname |
DISTINCT keyword
Select all the different genders
1 | SELECT DISTINCT sex |
Function
COUNT function
Find the number of employees
1 | SELECT COUNT(emp_id) |
Find the number of female employees born after 1970
1 | SELECT COUNT(emp_id) |
AVG function
Average of a column
Find the averge of all employee’s salaries
1 | SELECT AVG(salary) |
SUM function
Find the sum of all employee’s salaries
1 | SELECT SUM(salary) |
GROUP BY keyword
Find out how many males and females there are
1 | SELECT COUNT(sex), sex |
Find the total sales of each salesman
1 | SELECT SUM(total_sales), emp_id |
Wildcards
% is any number characters
_ is one character
LIKE keyword
Find any client’s who are an LLC
1 | SELECT * |
Find any branch suppliers who are in the label business
1 | SELECT * |
Find any employee born in October
1 | SELECT * |
Find any clients who are schools
1 | SELECT * |
UNION
Find a list of employee and branch names
1 | SELECT first_name |
Other uses:
1 | SELECT first_name AS Compaany_Names |
1 | SELECT client_name, branch_id |
or
1 | SELECT client_name, client.branch_id |
Find a list of all money spent or earned by the company
1 | SELECT salary |
JOIN
Combine rows from two or more tables based on related column
1 | SELECT employee.emp_id, employee.first_name, branch.branch_name |
LEFT JOIN:
Includes all the rows in employee table (result Null for not match)
RIGHT JOIN:
includes all the rows in branch table (result Null for not match)
FULL JOIN:
LEFT JOIN + RIGHT JOIN
Not in MySQL
Nested Queries
Use more SELECT
Find the names of all employees who have sold over 30,000 to a single client
1 | SELECT employee.first_name, employee.last_name |
Find all clients who are handled by the branch that Michael Scott manages. Assume given Michael’s ID
1 | SELECT client.client_name |
If use =, better limit the result to 1.
Delete entries when they have foreign key
ON DELETE
Foreign keys set to null
1 | CREATE TABLE branch ( |
ON DELETE CASCADE
Delete the entire row
Can be used when the deleting key is primary key since primary key cannot be null
1 | CREATE TABLE branch ( |
Trigger
In command prompt, type
1 | use student; |
1 | INSERT INTO employee |
NEW
Refer to the new row inserting
1 | DELIMITER $$ |
IF statement
1 | DELIMITER $$ |
Trigger for update, delete…
TRIGGER my_trigger BEFORE UPDATE
…
TRIGGER my_trigger BEFORE DELETE
…
AFTER
TRIGGER my_trigger AFTER UPDATE
…
DROP TRIGGER
DROP TIGGER my_trigger;
ER Diagram
by Mike Dane from mikedane.com
Schema
by Mike Dane from mikedane.com